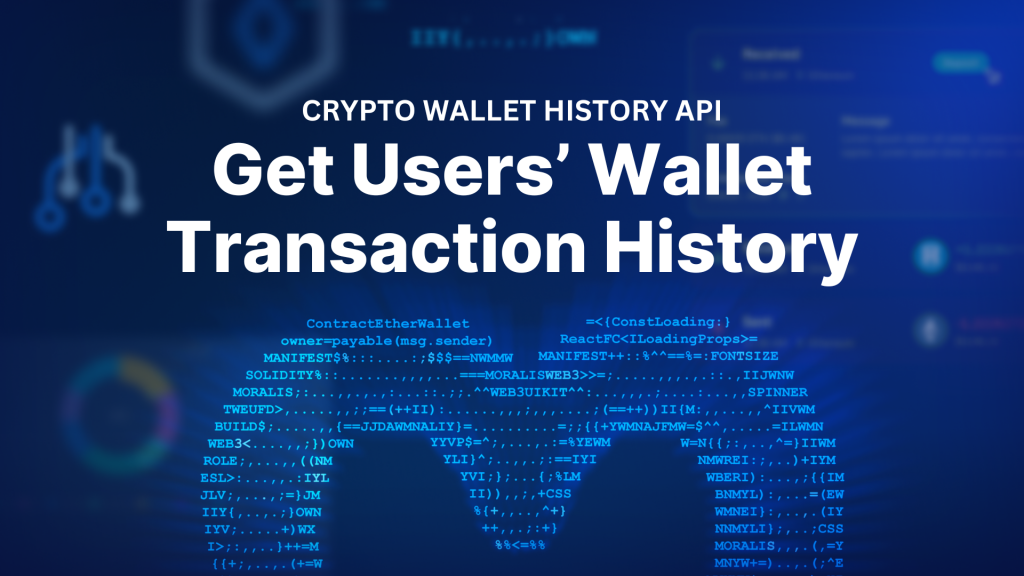
Building decentralized applications (dapps) and Web3 wallets requires access to historical data related to crypto addresses. However, querying this information without proper tools is easier said than done. Fortunately, it’s possible to simplify the process significantly using a crypto wallet history API, and your best option is Moralis’ industry-leading Wallet API!
With only single lines of code, you can use the Wallet API to get historical data from any crypto address. This includes everything from native transactions and ERC-20 transfers to token balances at any given time. To give you an example, here’s how easy it is to fetch a wallet’s native transaction history using the getWalletTransactions()
endpoint:
const response = await Moralis.EvmApi.transaction.getWalletTransactions({ "chain": "0x1", "address": "0x1f9090aaE28b8a3dCeaDf281B0F12828e676c326" });
If you want a more detailed explanation of how this works and what other endpoints you can call, join us in this article as we explore the ins and outs of Moralis’ Wallet API! Also, if you want to leverage this industry-leading tool yourself, don’t forget to sign up with Moralis. You can create an account for free and immediately start leveraging our premier suite of Web3 APIs!
Overview
In today’s article, we’ll start by exploring the ins and outs of crypto wallet history APIs. From there, we’ll introduce you to Moralis’ Wallet API – a premier tool that not only provides the functionalities of a crypto wallet history API, but also goes beyond that feature. So, as we progress, we’ll cover this tool’s most prominent features and provide some examples of endpoints that you’ll likely find helpful when building dapps. Next, we’ll dive into our main tutorial and show you how to get a user’s wallet transaction history in three steps:
- Get a Moralis API Key
- Write a Script Calling the
getWalletTransactions()
Endpoint - Run the Code
Lastly, to top things off, we’ll explore Moralis further and look closer at additional tools you can combine with the Wallet API to build sophisticated Web3 projects!
So, if you already know what a crypto wallet history API is and how it works, feel free to jump straight into the ”Introducing Moralis’ Wallet API…” section. Otherwise, join us below as we kick things off by dissecting what a crypto wallet history API is!
What is a Crypto Wallet History API?
Whether you’re building a decentralized exchange (DEX), portfolio tracker, Web3 wallet, or any other decentralized application (dapp), you’ll likely realize you need access to your users’ wallet histories. This includes everything from past balances to a complete record of all transactions!
But why exactly do you need this data?
To answer the query above, let’s use Web3 wallets as an example. Access to an address’ history is essential when building a Web3 wallet for numerous reasons. For one, it allows you to seamlessly display a user’s past transactions, which is a fundamental aspect of most crypto wallets. However, it additionally unlocks the potential for other prominent features, such as historical data analysis, transaction tracking, tax reports, etc.
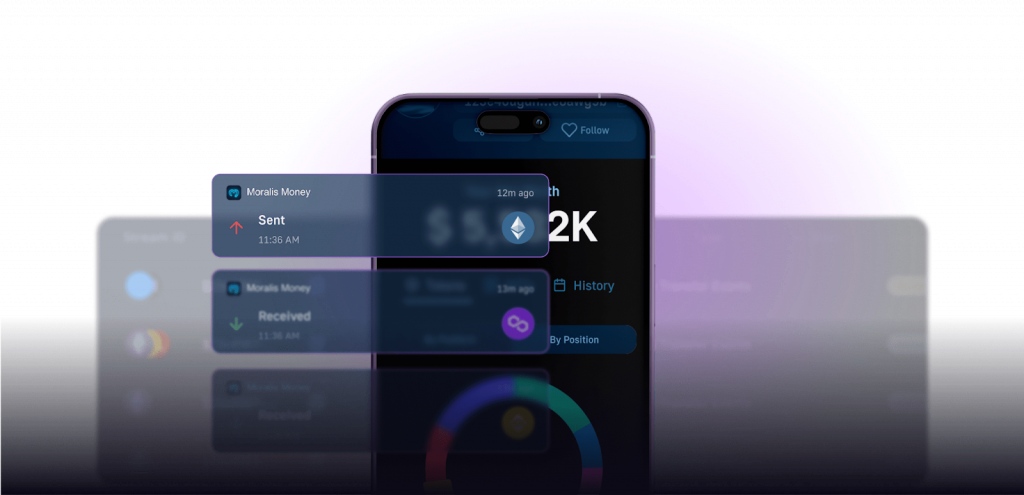
So, how can you get this data?
Well, from a conventional perspective, it has been quite a challenge to query a blockchain network for this information. And this is precisely why Web3 developers leverage crypto wallet history APIs to simplify the workflow!
But what is a crypto wallet history API?
A crypto wallet history API (application programming interface) is a set of methods, rules, and protocols allowing you to seamlessly interact with a blockchain network to integrate wallet functionality and historical data into your dapps. Essentially, a crypto wallet history API makes the process of fetching historical wallet data from a blockchain network easy. And with these interfaces, you can leverage premade protocols and methods, meaning you don’t have to reinvent the wheel. In return, you can save lots of development time and resources when building dapps!
So, which is the best crypto wallet history API?
For the answer to this question, join us in the next section as we introduce you to Moralis’ industry-leading Wallet API!
Introducing Moralis’ Wallet API – The Easiest Way to Get the History of a Crypto Wallet
Moralis’ Web3 Wallet API is the ultimate tool for building wallets and integrating wallet functionality into your dapps. The Wallet API boasts an extensive array of features, unparalleled scalability, and exceptional flexibility, allowing you to build Web3 projects that will blow the competition out of the water!
Our Wallet API supports over 500 million addresses and most major blockchain networks, including Ethereum, Polygon, Optimism, Arbitrum, BNB Smart Chain (BSC), and many others. Consequently, when working with Moralis, it has never been easier to build cross-chain compatible dapps.
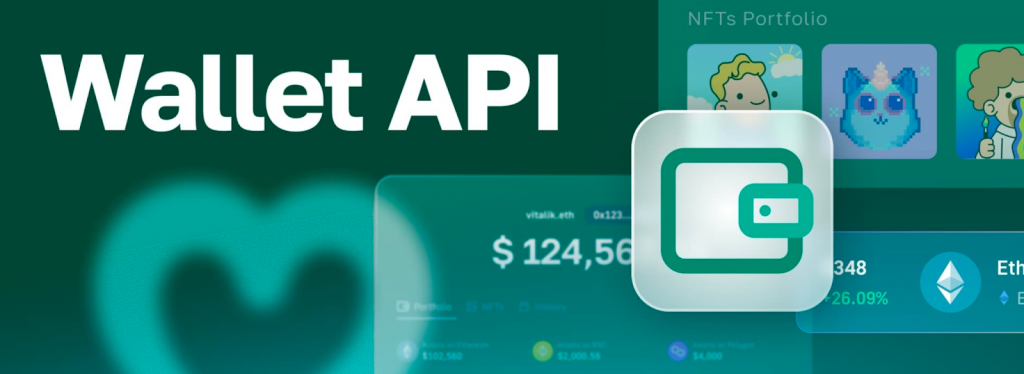
With only single lines of code, you can effortlessly get historical wallet data, including native transactions, token balances, NFT balances, transfers, and much more, for any wallet on any chain! Furthermore, not only does this premier interface provide all the essential crypto wallet history API functionality, but many additional features as well. And to highlight the full power of this industry-leading tool, let’s explore some prominent examples below:
- Historical Data: Get historical wallet history data across token transfers, native transactions, internal transactions, NFTs, and more.
- Real-Time Data: Fetch real-time token balances, NFT balances, transfers, etc.
- Decoded Transactions: Access decoded transactions so you can seamlessly connect the dots and understand what’s happening on the blockchain.
- Address Labels: All transfer and transaction endpoints are equipped with fully enriched address labels for public addresses, including Coninbase, Uniswap, 1inch, and many more.
- Profile Data: Get access to a wallet’s age, chain activity, etc.
- ERC-4337 Compatible: The Wallet API is ERC-4337 compatible. As such, it works perfectly with account abstraction, allowing you to effortlessly fetch token balances, transaction data, and transfers for any smart contract account.
Nevertheless, with an overview of Moralis’ Wallet API, let’s now explore some of our prominent endpoints for getting the history of a crypto wallet!
Moralis’ Wallet API Endpoints for Getting the History of a Crypto Address
In this section of the guide, we’ll explore three of the Wallet API’s most prominent endpoints for historical data. In doing so, we’ll show you how to fetch historical transactions and the native and ERC-20 balances of any wallet at any given point in time!
getWalletTransactions()
– Get an array of all native transactions of any wallet ordered by block number in descending order. All you need to do is call the endpoint while passing along two parameters:chain
andaddress
:
const response = await Moralis.EvmApi.transaction.getWalletTransactions({ "chain": "0x1", "address": "0x1f9090aaE28b8a3dCeaDf281B0F12828e676c326" });
getNativeBalance()
– Fetch the native balance of any wallet at any given point in time. Simply call the endpoint while passing along three parameters:chain
,toBlock
, andaddress
. ThetoBlock
parameter specifies from which block you want to fetch the data:
const response = await Moralis.EvmApi.balance.getNativeBalance({ "chain": "0x1", "toBlock": 18541416, "address": "0xDC24316b9AE028F1497c275EB9192a3Ea0f67022" });
getWalletTokenBalances()
– Query an array of tokens held by a wallet at any given point in time. All you have to do is call the endpoint while passing along three parameters:chain
,toBlock
, andaddress
. As in the previous example, thetoBlock
parameter specifies the block from which you want to get the data:
const response = await Moralis.EvmApi.token.getWalletTokenBalances({ "chain": "0x1", "toBlock": 18541416, "address": "0x1f9090aaE28b8a3dCeaDf281B0F12828e676c326" });
That covers our three examples. If you wish to explore other endpoints for historical data, check out our official Wallet API documentation page!
In the next section, we’ll give you a comprehensive tutorial on how you can call the endpoints above. So, if you’d like to learn more about this, join us below as we show you how to get the transaction history of a crypto address using Moralis’ Wallet API!
3-Step Tutorial: How to Get the Transaction History of a Crypto Address Using Moralis’ Wallet API
In this brief tutorial, we’ll demo the Wallet API by showing you how to get the native transaction history of a crypto address in three steps:
- Get a Moralis API Key
- Write a Script Calling the
getWalletTransactions()
Endpoint - Run the Code
However, you must deal with a few prerequisites before you can continue!
Prerequisites
The Wallet API and Moralis’ SDK work with multiple programming languages, including Python, TypeScript, etc. However, for this tutorial, we’ll be using JavaScript. As such, make sure you have the following ready before you move on:
Step 1: Get a Moralis API Key
To call the Wallet API, you need to have an API key. And to get one, you need a Moralis account. As such, start by signing up with Moralis and set up your first project!
Next, go to the ”Settings” tab, scroll down until you find the ”API Keys” section, and copy your Moralis API key:
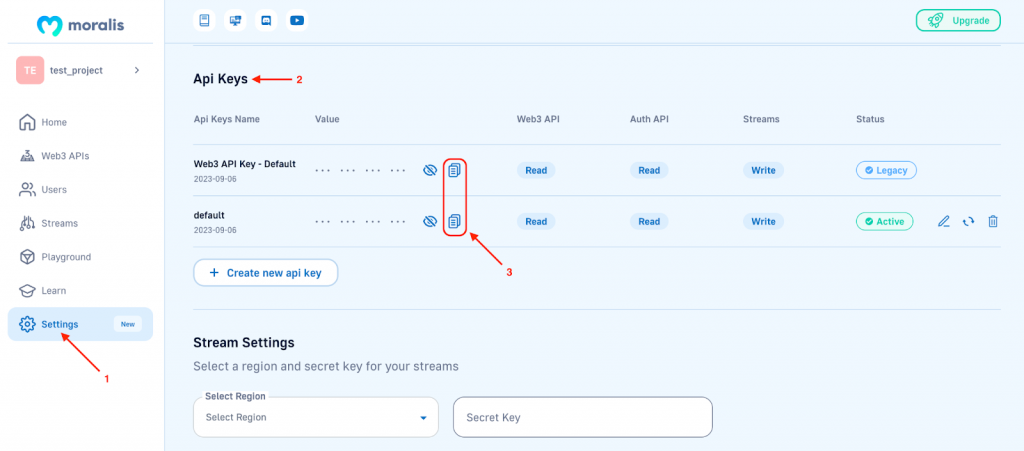
Keep the key for now, as you’ll need it in the next step to initialize the Moralis SDK!
Step 2: Write a Script Calling the getWalletTransactions()
Endpoint
Next up, start by setting up a new project in your preferred IDE. You can then run the following terminal command in the root folder to install the Moralis SDK:
npm install moralis @moralisweb3/common-evm-utils
From here, create a new ”index.js” file and add the following code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = "0x26fcbd3afebbe28d0a8684f790c48368d21665b5"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.transaction.getWalletTransactions({ address, chain, }); console.log(response.toJSON()); }; runApp();
Next, you need to make a few configurations. And you can start by replacing YOU_API_KEY
with the key you got during the first step:
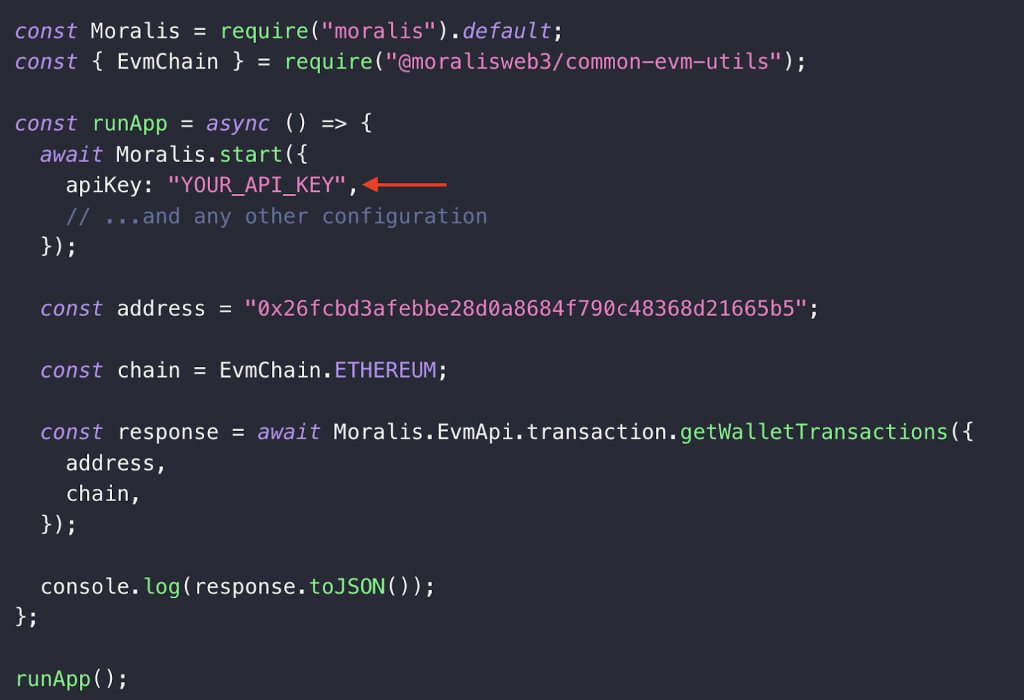
From here, configure address
and chain
to fit your query:
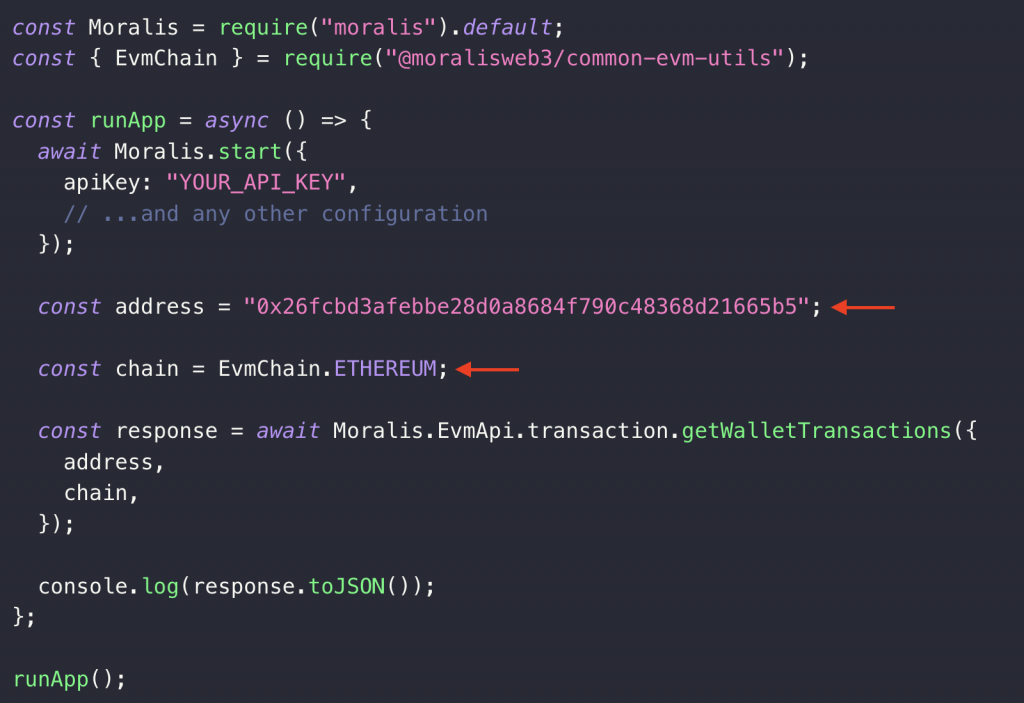
We then call the getWalletTransactions()
endpoint while passing along address
and chain
as parameters:
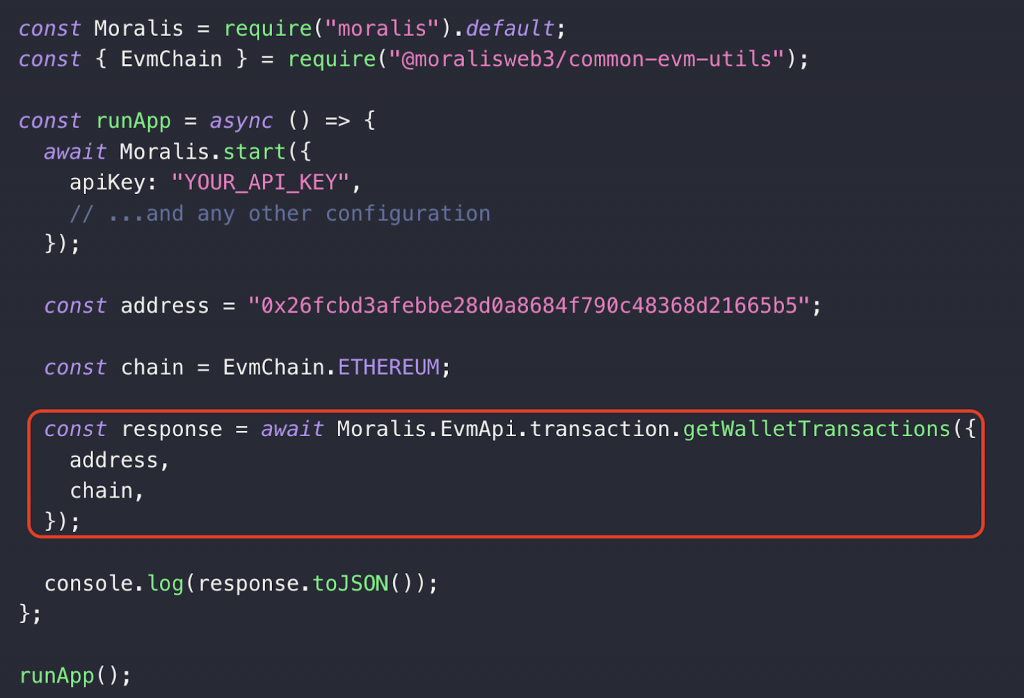
That’s it; all you need to do from here is run the code!
Step 3: Run the Code
For the final step, open a terminal and run this command in your project’s root folder to execute the script:
node index.js
In return for running the code, you’ll get an array of all historical native transactions from the specified wallet ordered by block number in descending order. Here’s an example of what it might look like:
//... "result": [ { "hash": "0x7f582e6b778a836f6d142fe235348ccb1a1320546038ee9025a4d4e74812aad9", "nonce": "368731", "transaction_index": "194", "from_address": "0xd4a3BebD824189481FC45363602b83C9c7e9cbDf", "to_address": "0xa71db868318f0a0bae9411347cd4a6fa23d8d4ef", "from_address_label": "Binance 1", "to_address_label": "Binance 2", "value": "66015740581568276", "gas": "21000", "gas_price": "46898567213", "input": "0x", "receipt_cumulative_gas_used": "15103012", "receipt_gas_used": "21000", "receipt_contract_address": null, "receipt_root": null, "receipt_status": "1", "block_timestamp": "2023-11-16T16:14:11.000Z", "block_number": "18585572", "block_hash": "0x12df791b56941267d162ae19ceaefabb73181fb02aa780f245b4398fac5c7e41", "transfer_index": [ 18585572, 194 ] }, //... ]
The response contains a bunch of information about each transaction, including basic data such as transaction hashes, transfer amounts, timestamps, gas used, etc.
However, it also includes other helpful information, such as labels for both the to and from addresses. With this information, you can effortlessly provide your customers with human-readable labels, significantly improving the user experience of your dapps:
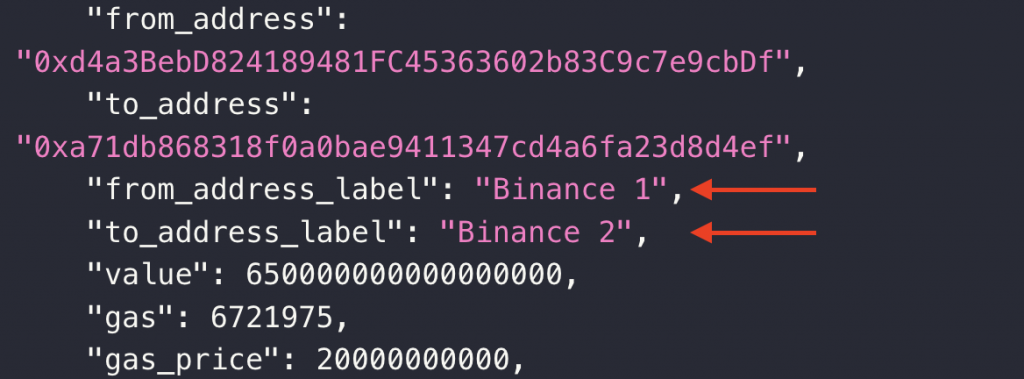
Congratulations; you now know how to use Moralis’ Wallet API to get the transactions of any crypto address!
Also, you can follow the same steps for any of our other endpoints. All you need to do is make minor code configurations during the second step!
Beyond the Wallet API – Exploring Moralis’ Web3 APIs Further
Moralis is the industry’s premier Web3 API provider, and our suite of enterprise-grade development tools makes Web3 development as seamless as Web2. Consequently, when working with Moralis, it has never been easier to build everything from NFT marketplaces to Web3 wallets!
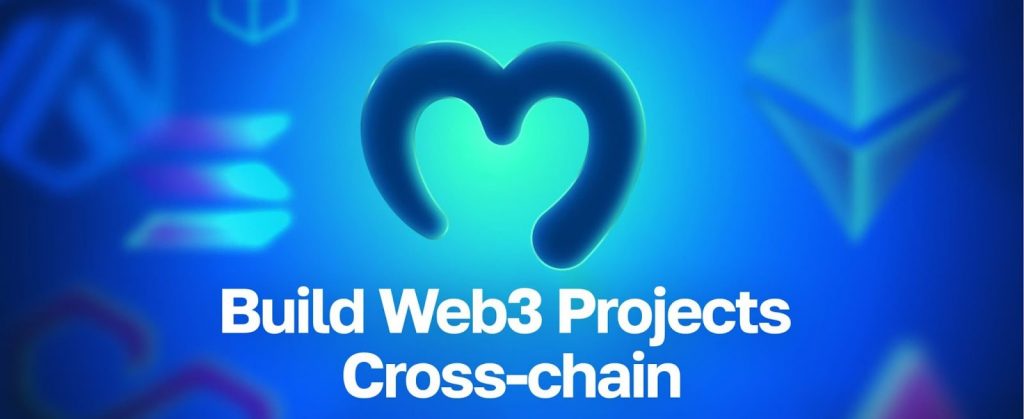
In addition to the Wallet API, Moralis also offers other development tools you’ll find useful when creating Web3 projects. And to highlight the power of our Web3 API suite, you’ll find three prominent examples below that are a perfect match for building dapps together with the Wallet API:
- NFT API: The NFT API is the industry’s leading tool for NFT data. With only single lines of code, you can effortlessly fetch NFT balances, metadata, real-time transfers, on-chain pricing data, etc.
- Token API: The Token API allows you to seamlessly get and integrate token data into your dapps. This includes real-time token prices, wallet balances, transfers, and much more.
- Streams API: The Streams API is the ultimate tool for monitoring on-chain events and setting up real-time alerts. With this interface, you can set up customized streams to get on-chain events sent directly to your project’s backend via Web3 webhooks in real time. As such, this is the perfect tool for building things such as an on-chain wallet tracker!
Also, all of Moralis’ Web3 APIs are fully cross-chain compatible. This means that you can use our tools to build dapps on multiple blockchain networks, including Ethereum, Polygon, BSC, etc.
However, the tools mentioned above are only a few examples of interfaces that work perfectly together with the Wallet API. If you want to explore all our tools, check out our official Web3 API page!
Summary: Exploring the #1 Crypto Wallet History API
In today’s article, we introduced you to Moralis’ Wallet API – the ultimate tool providing the full functionality of a crypto wallet history API and much more. In doing so, we learned that you can use this tool to fetch historical wallet data, including everything from transactions to token balances, at any given point in time.
What’s more, we also highlighted the power of the Wallet API by showing you how to get a user’s crypto transaction history in three straightforward steps:
- Get a Moralis API Key
- Write a Script Calling the
getWalletTransactions()
Endpoint - Run the Code
As such, if you have followed along this far, you now know how to use the Wallet API to get historical data from any wallet on any blockchain network. With your newly acquired skills, you can easily build a Web3 wallet or integrate wallet functionality into your projects!
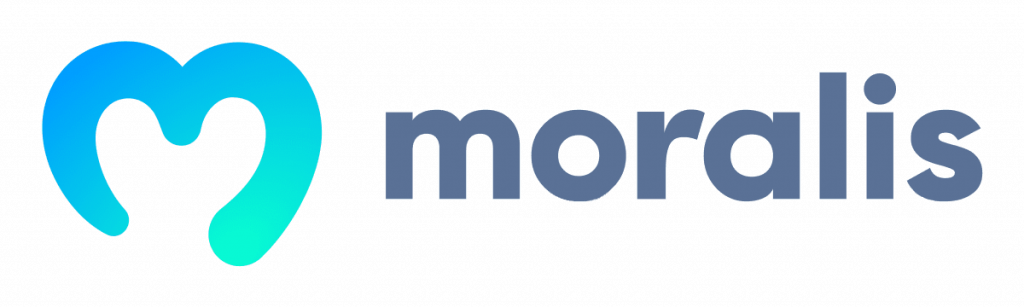
If you found this crypto wallet history API tutorial interesting, check out other guides here at Moralis. For instance, read about the top block explorer API or learn more about the Gnosis API! Also, if you want to use the Wallet API in your development endeavors, don’t forget to sign up with Moralis!
Read More: moralis.io